HackerRank Delete a Node from a Linked List | JS Solution
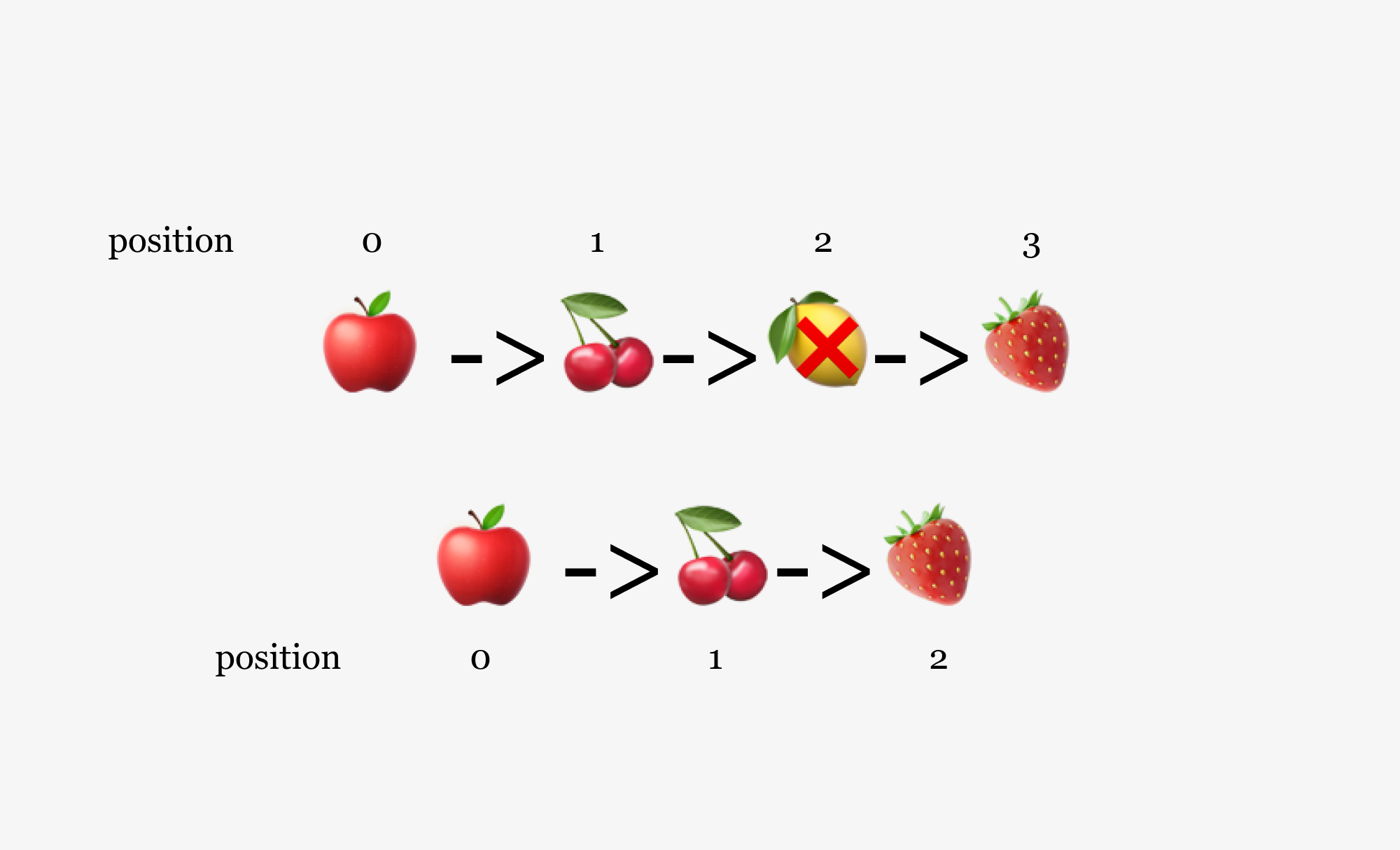
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
param {Node} head
param {number} position
return {Node} head
*/
Test Case


JavaScript Solution
function deleteNode(head, position) {
if (!head) {
return null;
}
let nodeToDelete = head;
if (position === 0) {
head = nodeToDelete.next;
return head;
}
let count = 0;
let prev = null;
while (nodeToDelete) {
if (count === position) {
console.log(count);
prev.next = nodeToDelete.next;
return head;
}
prev = nodeToDelete;
nodeToDelete = nodeToDelete.next;
count++;
}
return null;
}
Algorithm Source
Delete a Node Algorithm by HackerRank