HackerRank Dictionaries and Maps | JS Solution
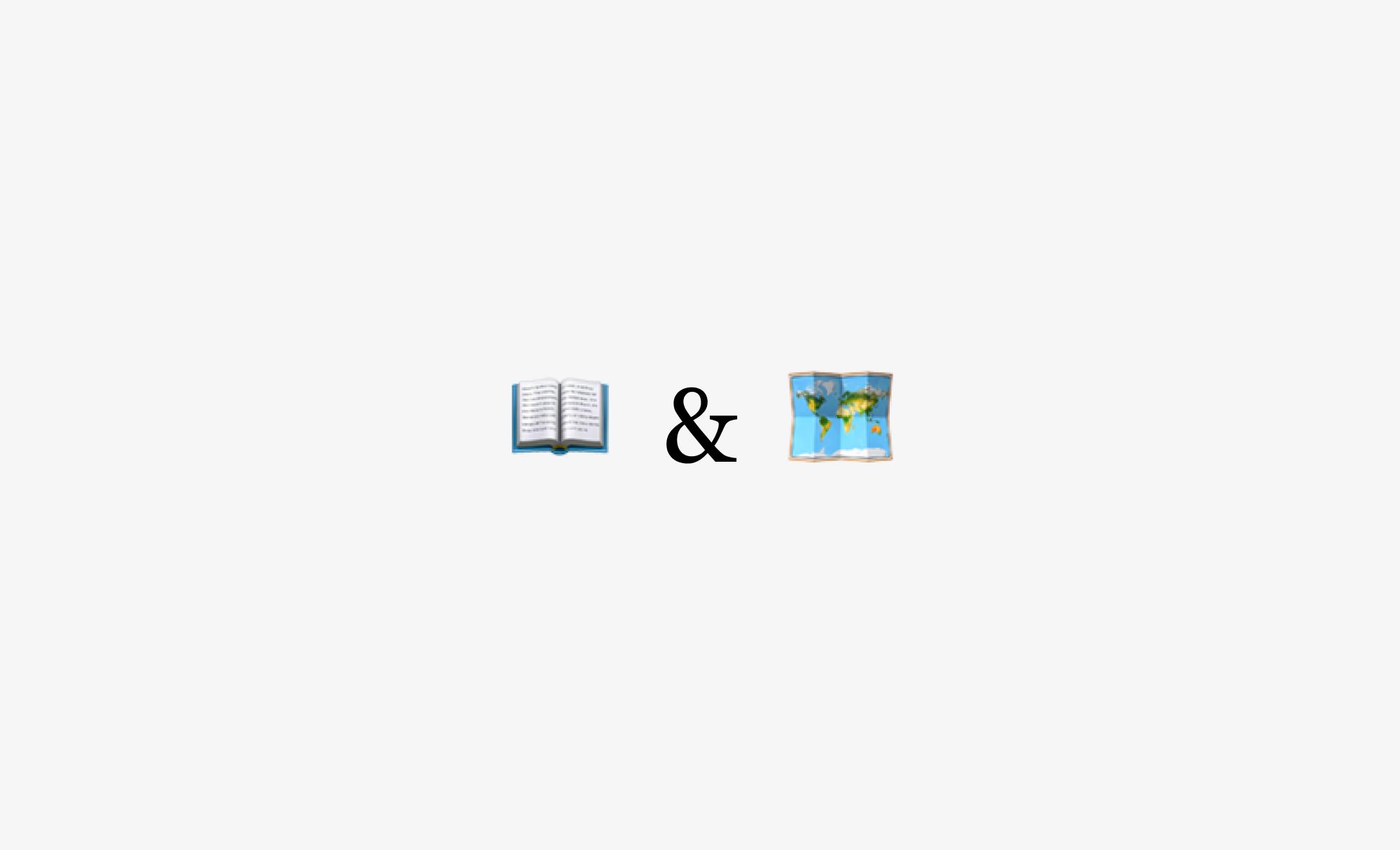
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
input {string} '3\nsam 99912222\ntom 11122222\nharry 12299933\nsam\nedward\nharry'
- 1st line: `n` number of entries in the phone book
- `n` lines: `name phoneNumber` 2 space-separated values on a single line
- unknown number of lines: `name` queries
output {string} 'key=value' or 'Not found'
*/
Test Case
input = '3\nsam 99912222\ntom 11122222\nharry 12299933\nsam\nedward\nharry';
Pseudocode
- Let's first validate the
input
- Then, process that
input
, so that we have 3 data points separated: 1) number of entries in the phone book, 2) entries, and 3) queries - Next, let's create an object representation of the phone book with all of the provided entries
phoneBookObj
- Lastly, we go ahead and run through the queries to see if they exist in our phone book and either output the query in
'key=value'
format or'Not found'
JavaScript Solution
function processData(input) {
if (!input || typeof input !== 'string') {
throw new Error('`input` must be a `string` data type');
}
let arr = input.split('\n');
let entriesArr = arr.slice(1, parseInt(arr[0]) + 1);
let queriesArr = arr.slice(parseInt(arr[0]) + 1);
let phoneBookObj = {};
entriesArr.forEach(contact => {
let entry = contact.split(' ');
phoneBookObj[entry[0]] = entry[1];
})
queriesArr.forEach(query => {
if (phoneBookObj[query]) {
console.log(`${query}=${phoneBookObj[query]}`);
} else {
console.log('Not found');
}
})
// console.log('entriesArr: ', entriesArr);
// console.log('queriesArr: ', queriesArr);
// console.log('phoneBookObj: ', phoneBookObj);
};
Here are some test logs that show what our variables created above look like:
entriesArr: [ 'sam 99912222', 'tom 11122222', 'harry 12299933' ]
queriesArr: [ 'sam', 'edward', 'harry' ]
phoneBookObj: { sam: '99912222', tom: '11122222', harry: '12299933' }
Resources
- Dictionaries and Maps algorithm by HackerRank
- .split(), .slice() and .forEach() methods by MDN Web Docs
- parseInt() function by MDN Web Docs