HackerRank Operators | JS Solution
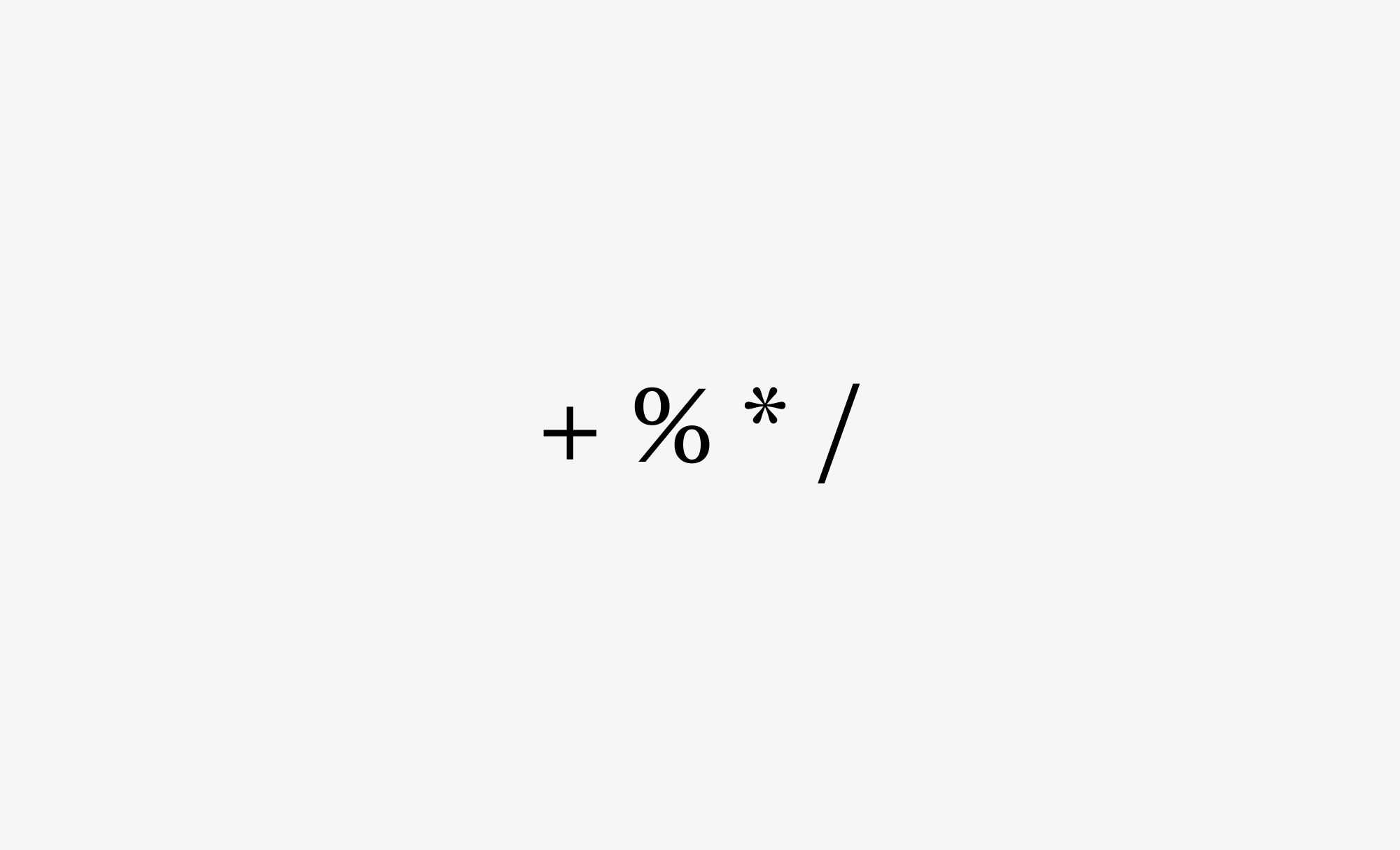
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/**
* Calculate and print rounded to the nearest integer result.
* @param {number} meal_cost
* @param {number} tip_percent
* @param {number} tax_percent
* @print {number} result
* @return {undefined}
*/
Test Case
meal_cost = 15.00;
tip_percent = 20;
tax_percent = 13;
solve(15.00, 20, 13); // prints 20
JavaScript Solution
function solve(meal_cost, tip_percent, tax_percent) {
let result = meal_cost + (meal_cost * tip_percent / 100) + (meal_cost * tax_percent / 100);
console.log(Math.round(result));
}
Resources
- Operators algorithm by HackerRank
- Math.round() method