HackerRank Repeated String | JS Solution
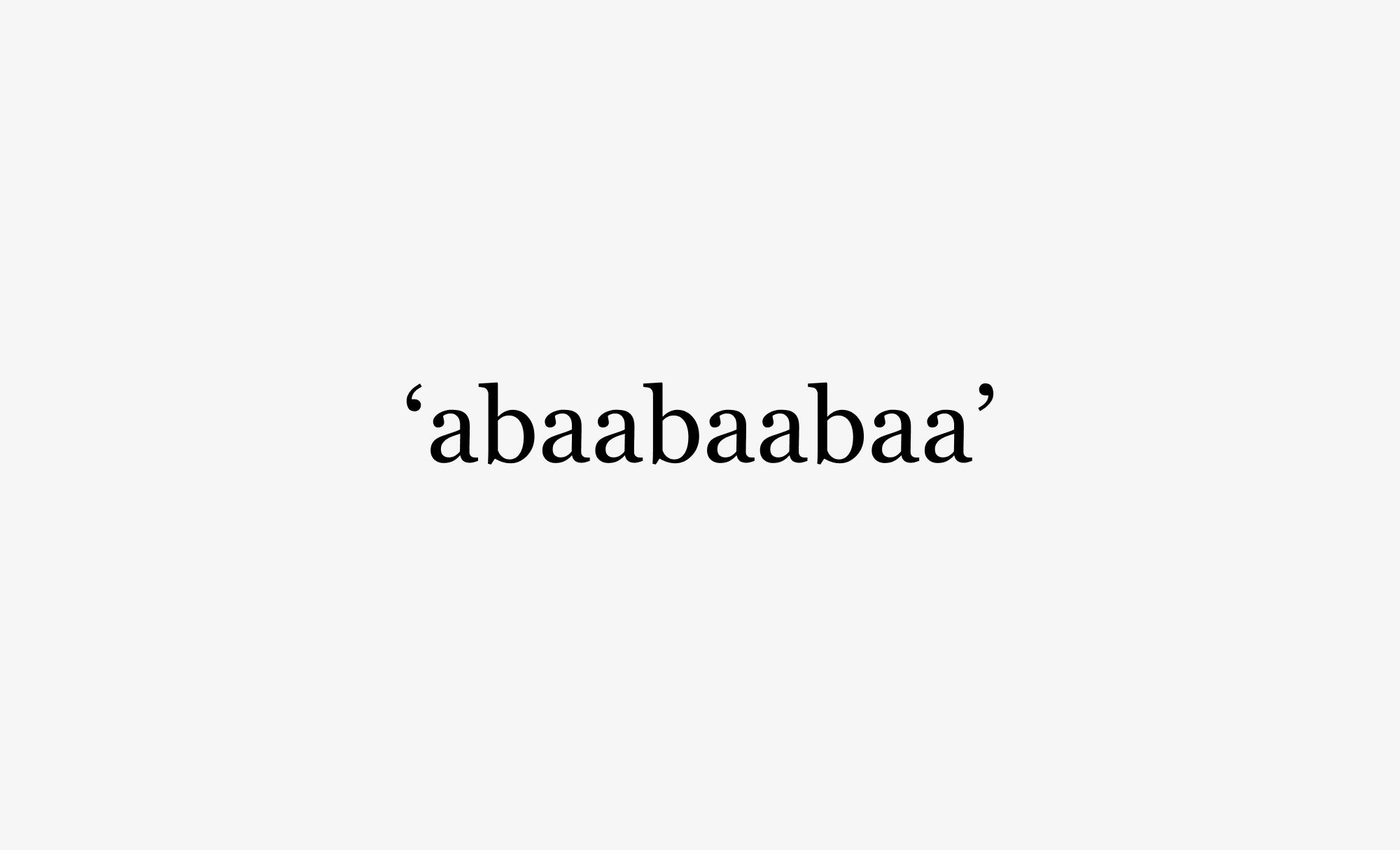
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
param {string} s
param {number} n
returns {number} Number of `a` occurances
*/
Test Cases
repeatedString('aba', 10);
repeatedString('a', 1000000);
Pseudo Code
- Let's figure out how many times the string
s
will be repeated in our algorithm without remainder and record the number infullRepeats
variable. This allows us to skip through inefficient looping over the same strings
.
For example, if we start with the following inputss = 'aba', n = 10
, we can see that'aba'
string will be repeated 3 times in full in our function ('aba-aba-aba-a'
) and therefore we can only count'a'
s in'aba'
once and then multiply the result by the number of full string repeatscountA = countA * fullRepeats
or2 * 3 = 6
- Now, if there was a remainder, we can loop over our sting
s
one more time up to thatremainder
- Lastly, let's return the
countA
JavaScript Solution
function repeatedString(s, n) {
let fullRepeats = Math.trunc(n / s.length);
let remainder = n % s.length;
let countA = 0;
for (let i = 0; i < s.length; i++) {
if (s.charAt(i) === 'a') {
countA++;
}
}
countA = countA * fullRepeats;
if (remainder) {
for (let i = 0; i < remainder; i++) {
if (s.charAt(i) === 'a') {
countA++;
}
}
}
return countA;
}
Resources
- Repeated String algorithm by HackerRank
- Math.trunc() and charAt() by MDN Web Docs