HackerRank Insert a Node at a Specific Position in a Linked List | JS Solution
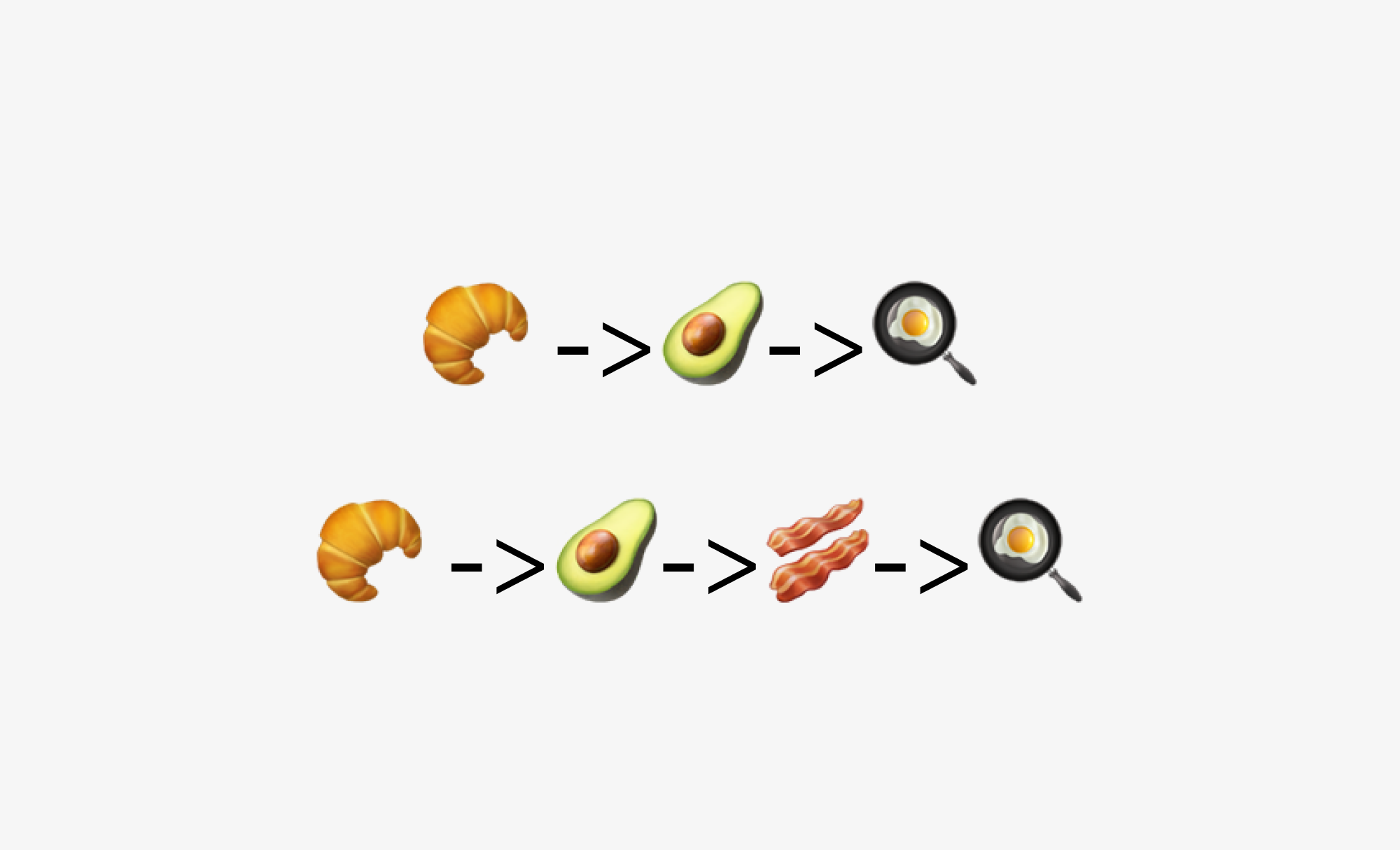
Problem
HackerRank detailed problem description can be found here.
We need to create a function insertNodeAtPosition(head, data, position) {}
. This function should:
- Create a new node using provided
data
- Insert that newly created node at the provided
position
inside a Singly Linked List - Lastly, return the
head
node
Inputs & Outputs
/*
param {Node} head
param {number} data
param {number} position
return {Node}
*/
Test Case
I am using data
of a string data type in the example below in order to make this algorithm just a bit more visual, though in the actual coded solution the data
of a number data type will be used.
Imagine this is our initial linked list with 3 nodes in it. We are asked to create a new node with data bacon
and insert it in the position: 2
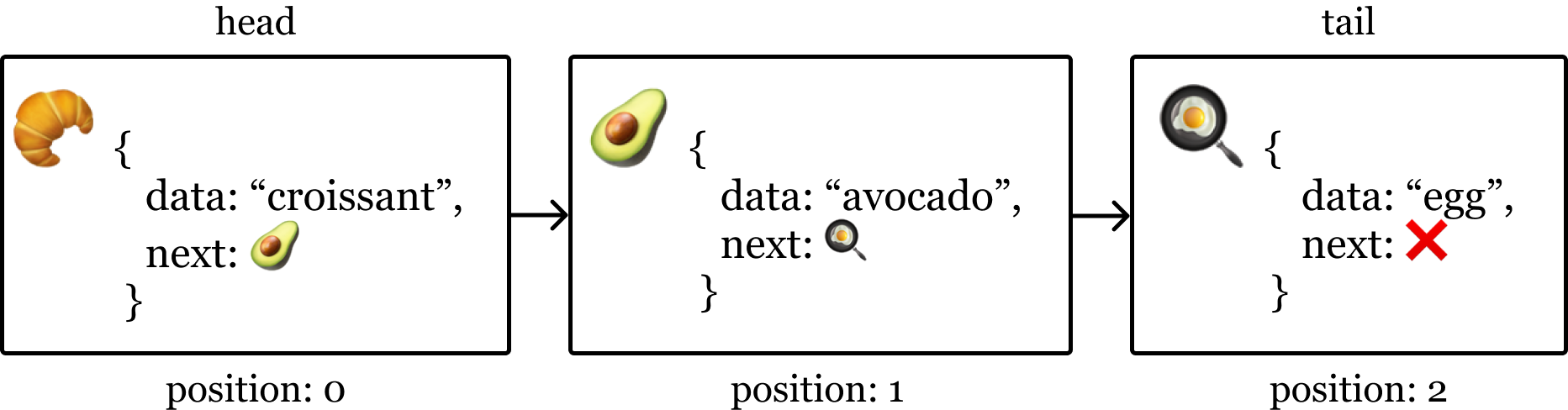
This is how our linked list would look like after we are done with our function:

Data Structures
Singly linked list, number
Code
function insertNodeAtPosition(head, data, position) {
let count = 0;
let prev = null;
let current = head;
let newNode = new SinglyLinkedListNode(data);
while (count < position && current) {
prev = current;
current = current.next;
count++;
}
if (prev) {
prev.next = newNode;
newNode.next = current;
}
return head;
}
* The code above doesn't consider the cases where we are given an empty linked list or position: 0.
Sources
Insert a node at a specific position in a linked list algorithm by HackerRank