What is a Stack Data Structure | JavaScript
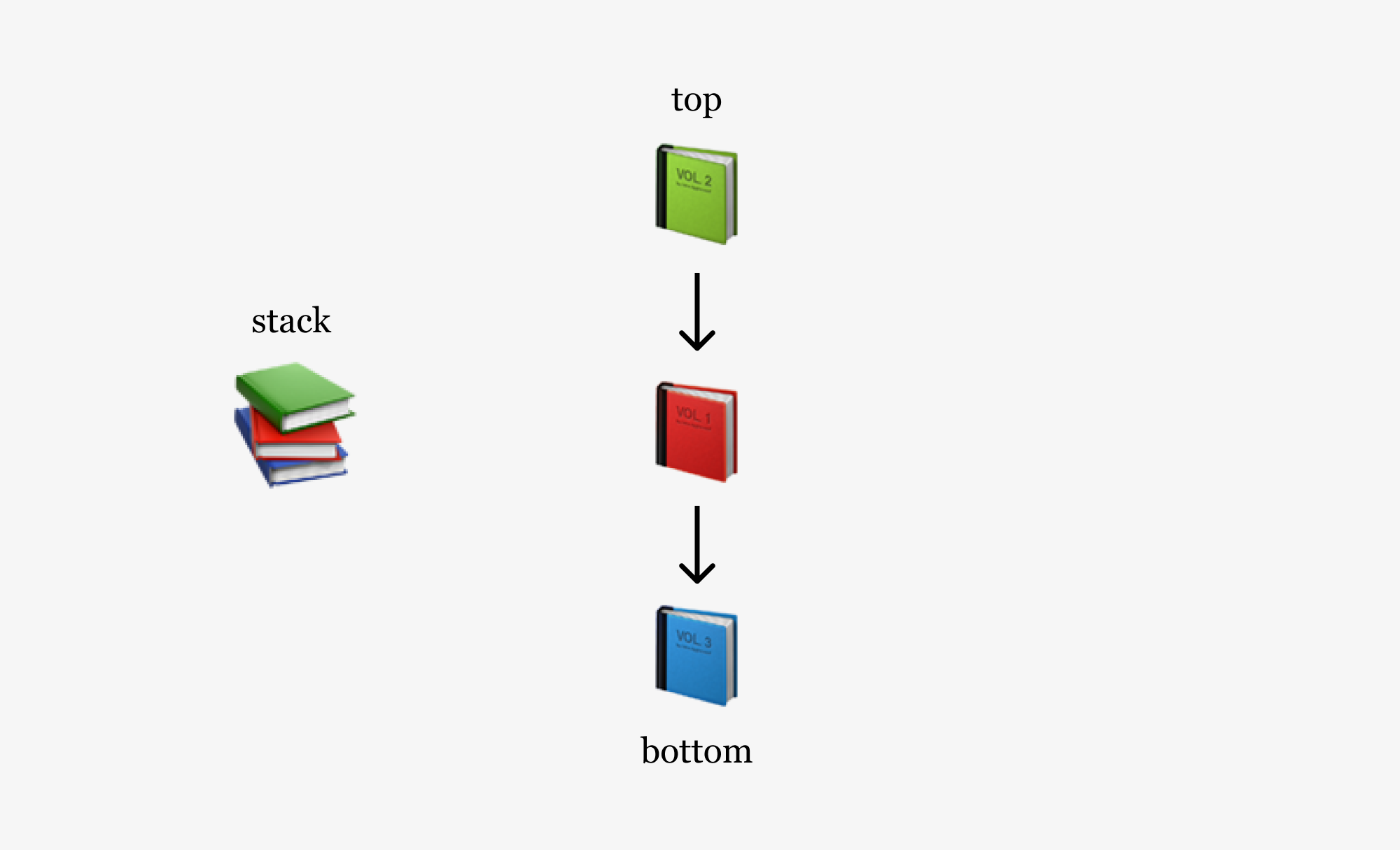
Stack is a last in, first out (LIFO) data structure in computer science. Stack is a linear collection of ordered data (nodes).
Stacks
- push - add data to the top of the stack (first/head node)
- pop - remove and return data (first/head node) from the top of the stack
- peek - return data from the top of the stack (first/head node)
- underflow - happens when we are trying to remove (pop) data from an empty stack
- overflow - happens when we are trying to add (push) data to the full stack
Data Types & Structures
Stacks are generally implemented using Linked Lists or Arrays.
Stack implementation using a Linked List
class Stack {
constructor(maxSize = Infinity) {
this.stack = new LinkedList();
this.size = 0;
this.maxSize = maxSize;
}
hasRoom() {}
isEmpty() {}
push(value) {}
pop() {}
peek() {}
}